QYay! App
For your homework, you will be working individually to create a live Q&A app. The main purpose of this assignment is to allow you to create a simple full-stack software application from scratch. You are free to choose any software stack and platform for development. However, there are some general requirements for the project.
General Requirements
- Your code must be in the GitHub repository provided for this homework.
- You are not required to deploy your application to production, but it should be runnable locally.
- The repository's
README
should include detailed instructions on how to set up and run your application in a local development environment. - In the
README
, you should also explain how your code works, what stack it uses, and any challenges you faced or features that are not implemented. It should provide comprehensive documentation of the basic functions of your app. - It is important to follow good coding practices, including modularizing your code and writing readable code.
- Your app must implement all the "must-have" features described in the appendix on this page. Additionally, you should work incrementally towards completing the homework and make weekly submissions as outlined in the attached project plan.
- Your app must persist data. It should have a database and ideally conform to the client-server architecture.
Starter Code
- Accept the invitation link provided to get access to the starter code.
- Clone this repository locally to begin your work.
Ensure the repository remains private. Do not change its visibility or add collaborators.
Appendix: Requirement Specification
Problem Statement
Organizers of live events often face challenges when it comes to managing audience questions during Q&A sessions. Traditional methods, such as passing around a microphone or selecting individuals to speak, can be time-consuming and exclude some attendees' questions. A solution is needed to streamline the Q&A process and ensure that all audience questions are acknowledged and answered.
Proposed Solution
The QYay! app will address this issue by providing a platform for event organizers to collect and manage audience questions during Q&A sessions. Organizers can create an event on the app and share it with the audience using a unique link or code. Attendees can join the event and submit questions anonymously, without requiring a login, throughout the live session. Additionally, participants can view a real-time list of submitted questions and upvote the ones they want to hear answered. Organizers have access to the same list and can respond to questions, marking them as answered.
Potential Clients
Event organizers who aim to streamline their Q&A process and ensure that all audience questions are acknowledged and addressed.
Functional Requirements
Must-Have
- As an event organizer, I want to create an event on the app, so that I can collect questions from the audience.
- As an attendee, I want to join the event using a unique link or code, so that I can submit my questions.
- As an attendee, I want to submit questions anonymously, so that I can freely ask questions without any privacy concerns.
- As an attendee, I want to view and upvote questions submitted by others, so that the most popular questions can be identified.
- As an organizer, I want to view a real-time list of questions, so that I can manage and respond to them effectively during the event.
Nice-to-Have
- As an organizer, I want to filter and sort questions, so that I can prioritize them based on popularity or relevance.
- As an attendee, I want to edit or delete my submitted questions, so that I can correct mistakes or change my mind.
- As an organizer, I want to mark questions as answered, so that the audience knows which questions have been addressed.
Non-Functional Requirements
Must-Have
- Usability: The user interface must be intuitive and easy to navigate for both organizers and attendees.
- Security: Ensure data privacy and protect against unauthorized access, especially considering the anonymous nature of submissions.
- Reliability: The system must ensure uptime during live events, with minimal downtime.
Nice-to-Have
- Performance: The app should handle simultaneous submissions and interactions smoothly, without significant delays.
- Scalability: The system should be able to accommodate large numbers of users as the client base grows.
- Accessibility: The app should be accessible to users with disabilities, adhering to standard accessibility guidelines.
Similar Existing Applications
Appendix: Example Use Case
Here's a use case for the user story: "As an event organizer, I want to create an event on the app, so that I can collect questions from the audience."
Title: Create Event for Q&A Session
Actors: Event Organizer
Preconditions: The event organizer must have an account on the QYay! app.
Flow:
- The event organizer logs into their account on the QYay! app.
- The organizer selects the “Create Event” option on the app's main interface.
- The app displays the event creation form.
- The organizer fills out the event creation form, which includes details such as the event name, description, date, time, etc.
- After filling in the details, the organizer submits the form.
- The QYay! app processes the information and creates the event.
- The app displays a confirmation page with the event details and provides an option to share the unique link or code with attendees.
Postconditions:
- The event is created on the QYay! app.
- The event organizer can view (and manage) the event (and the submitted questions.)
Exceptions:
- If any required information in the event creation form is missing or invalid, the app will display an error message prompting the organizer to correct the information.
- If there is a system error or connectivity issue during the submission, the app will notify the organizer to try submitting the form again.
This use case outlines the process an event organizer would follow to create a new event for a Q&A session using the QYay! app, along with handling potential exceptions.
While you are not required to write use cases for this homework, I would recommend it as it helps to guide the implementation and ensure that all the required features are properly addressed.
Appendix: Example Sequence Diagram
To create a sequence diagram for the user story "As an event organizer, I want to create an event on the app, so that I can collect questions from the audience," we'll assume the presence of a client (which could be a mobile app or web app) and a server, with the server further divided into API and Database components.
Here is the sequence diagram:
This diagram illustrates the interactions between the event organizer, the client application, the server API, and the database during the event creation process, including handling of errors and exceptions.
Although creating sequence diagrams for this homework is not required, I recommend it. Sequence diagrams help identify interactions and data flow between different components of your app, such as the frontend, backend, and the database.
Appendix: Example Wireframe
Here is a series of wireframe images representing the flow for the user story "As an event organizer, I want to create an event on the app, so that I can collect questions from the audience." These wireframes visually depict the key screens and interactions described in the use case:
- Login Screen: This screen includes fields for username and password, along with a login button.
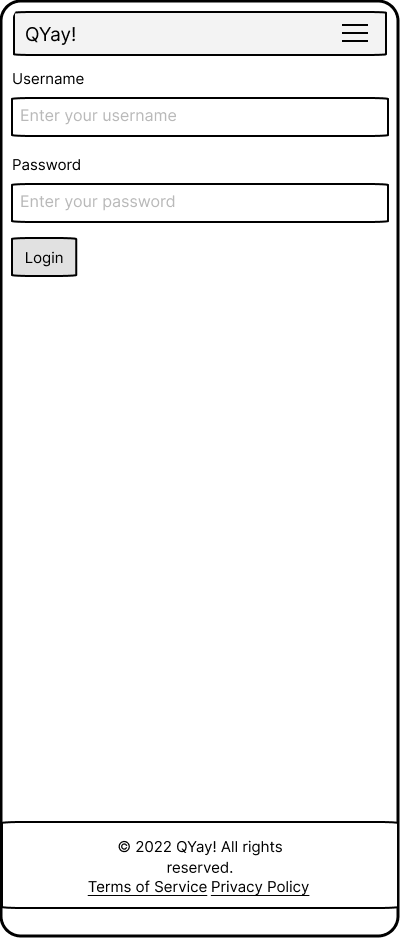
- Main Interface: Displays an option or button for "Create Event."
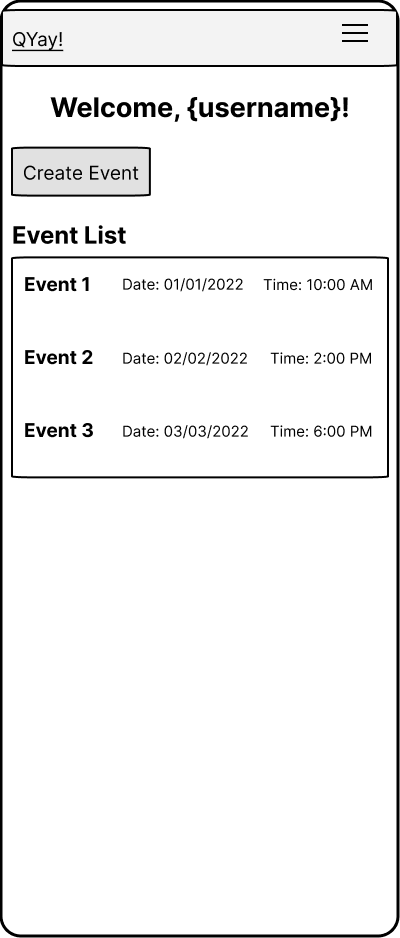
- Event Creation Form: Contains fields for the event name, description, date, time, along with a submit button.
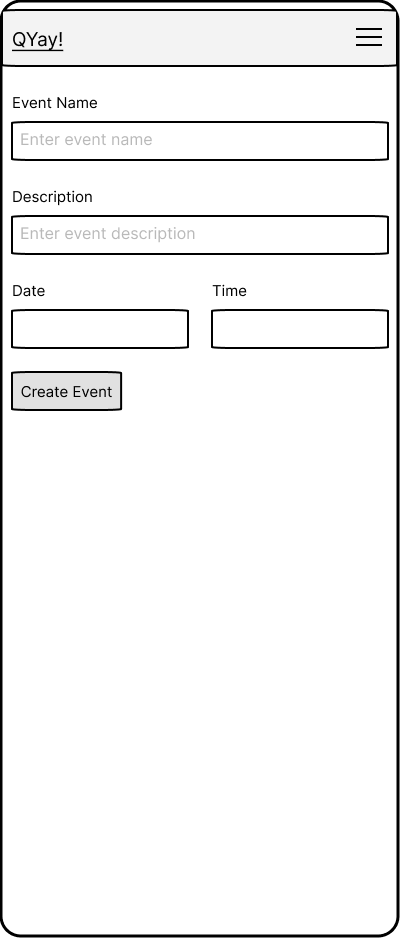
- Confirmation Page: Shows the details of the event that was created and provides an option to share the unique link or code.
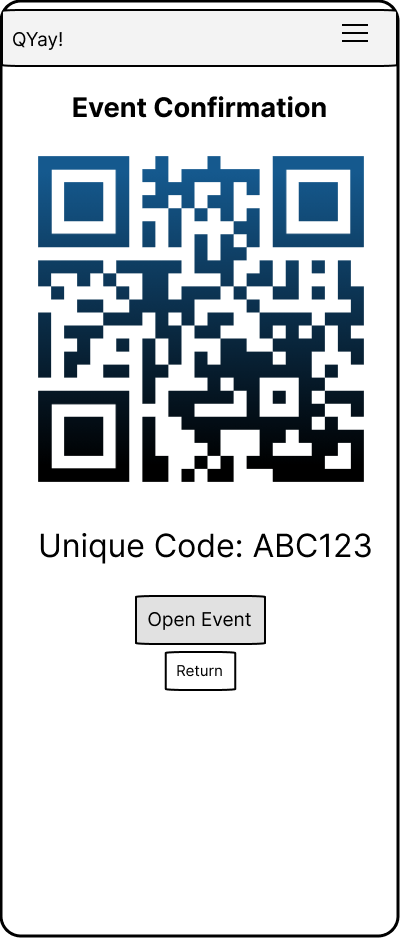
These wireframes are designed to be simple and schematic, typical for early-stage design and development.
Although creating wireframes for this homework is not required, I recommend it as it helps clarify what the frontend entails and what views are needed for a feature to be fully realized.
Appendix: Project Plan
Iteration 1
- Deadline: Saturday, Jan 27, at 11:00 PM ET
- Tasks:
- Clone the starter code
- Decide if you will build a web or mobile app
- Decide on your tech stack
- Create a Hello World app in your chosen tech stack
- Update the README with information on what stack you are using, and instructions to run the Hello World app locally
- Submit your work
- Notes:
- When I mention a "Hello World" app, I'm referring to creating a basic application in your chosen stack that simply displays a message like "Hello World". However, it should include all the components of your stack, such as the UI, backend, database, etc. This will help you confirm that your development environment is properly set up and that you can run and test code successfully.
- While you have the option to get ahead of schedule and accomplish more, please ensure that your submission includes all the required tasks for this iteration.
Iteration 2
- Deadline: Saturday, Feb 3, at 11:00 PM ET
- Tasks:
- Implement this user story: As an event organizer, I want to create an event on the app, so that I can collect questions from the audience.
- Please note that this user story, along with the non-functional requirements and app description, implies that event organizers must authenticate first. Therefore, your app should allow users to sign up and sign in. It is assumed that users who authenticate are event organizers, and they should have the ability to create an event.
- Update the README with instructions on how to run and test your app locally. Explain the features that were implemented, the difficulties and challenges you faced, and the learning resources you used.
- Submit your work
- Implement this user story: As an event organizer, I want to create an event on the app, so that I can collect questions from the audience.
Iteration 3
- Deadline: Saturday, Feb 10, at 11:00 PM ET
- Tasks:
- Implement the following user stories: (Note that the audience is not required to sign up/in.)
- As an attendee, I want to join the event using a unique link or code, so that I can submit my questions.
- As an attendee, I want to submit questions anonymously, so that I can freely ask questions without any privacy concerns.
- Update the README with instructions on how to run and test your app locally. Explain the implemented features, the difficulties and challenges you faced, and the learning resources you used.
- Submit your work
- Implement the following user stories: (Note that the audience is not required to sign up/in.)
Iteration 4
- Deadline: Saturday, Feb 17, at 11:00 PM ET
- Tasks:
- Implement the following user stories:
- As an attendee, I want to view and upvote questions submitted by others, so that the most popular questions can be identified.
- As an organizer, I want to view a real-time list of questions, so that I can manage and respond to them effectively during the event.
- Implement one of the nice-to-have user stories: you are free to choose any of them.
- Update the README with instructions on how to run and test your app locally. Explain the implemented features, the difficulties and challenges you faced, and the learning resources you used.
- Submit your work
- Implement the following user stories: